Programming Online Questionnaires with JavaScript and Java
Summary
1. Introduction
Within the last few years, the increasing proliferation of home computers coupled with a widespread use of the Internet and especially the World Wide Web (WWW) has shown to be of major importance for economic and social changes in the near future. The monitoring of these processes represents a challenge for social and market research. One of the most evident approaches for solving this task is to use the Internet itself for data collection. Various techniques have been developed to gather information from and about Internet users, among them e-mail surveys, chat groups and the observation of newsgroups (Romppe 1996, Batinic 1996). The most attractive part of the Internet, the World Wide Web, allows not only for a cost-efficient and timely distribution of information, it can also be applied to gather information via web-forms used as questionnaires for survey research.
The purpose of this paper is to investigate and present some of the potential of interactive HTML-forms (Hypertext Markup Language) as an environment for online research and primary data collection. This paper focuses on "client-side" interactivity as opposed to interactivity provided by a web-server via network transmissions of data. The programming languages JavaScript and Java allow for this client-side interactivity. The readers are assumed to have a general knowledge of the use of Hypertext Markup Language (HTML), especially its form elements, and of JavaScript and Java. This paper is not supposed to be a full tutorial on JavaScript or Java. For a brief introduction to HTML forms and examples of their use as questionnaires please refer to Batinic (1996) and Jacobs (1996). Documentation of JavaScript and Java is available at the web sites of Netscape and Sun.
2. Some Important Requirements for Online Questionnaires
Not only are the still unresolved issues of representative sampling and technical limitations of particular interest to the success or failure of a given survey but also the factors concerning the willingness of the potential respondent to participate.
Conceptually, the research design of an online survey closely resembles a mail survey with a self-administered questionnaire. For mail surveys it has proved to be useful to minimize the psychological and actual costs and to maximize the outcome for the respondent (for an overview see Hippler 1988). In other words: the principle of "give and take" use in successful web sites also seems to be applicable to online questionnaires.
The following requirements should be taken into consideration:
- to provide sufficient information about the research project and the institution conducting it as well as to explain the relevance of the respondent's participation.
- to explain the use of the questionnaire.
- to select an attractive, clear and ideally self-explaining layout for the questionnaire and its elements. For online questionnaires this also means the use of standard windows environment elements (e.g. checkboxes, radiobuttons).
- to enable the respondent to easily skip to the next relevant question (in the case of filters).
- to explicitly offer non-opinion alternatives ("Don't Know") and open-ended response alternatives ("Others", comments) to avoid forced choice situations.
- to break down long and complex questionnaires into smaller, more manageable parts.
- to minimise the respondent's expenses for telecommunication and online services and, as a consequence, to reduce or ideally avoid time pressure for the respondent.
Of great importance for the researcher is the requirement that the size and the complexity of the questionnaire and its questions are not (significantly) restricted by the applied technology and at least some of the circumstances of the interview are controllable. Specifically:
- under self-administered conditions the respondent is usually able to browse back and forth through the questionnaire. While the meaning of an ambiguous question may be clarified from its context, it is sometimes more advisable to control for the sequence and to limit the range of questions visible to the respondent.
- to control for question and item order effects, the technique used should allow for a random presentation of questions and items.
- to allow for a dynamic creation of questions depending on answers to previous questions.
- to collect additional information concerning date and time of the interview, answering time for selected questions and system variables such as browser type or operating system.
Last but not least, technical and economic aspects have to be considered, such as:
- maximum platform and browser independence of the system used.
- time and money invested into questionnaire programming.
- reliability of the questionnaire program.
3. Examples
Let's turn to the more technical aspects. For most of the above requirements client-side programming with JavaScript or Java can solve or at least reduces some of the emerging problems.
Java and JavaScript are general, object-oriented programming languages specially designed for the use on the WWW. Despite their very similar name they are quite different from each other. While both can be used to create interactive "content" in web pages, and both use a quite similar syntax, JavaScript is an interpreted scripting language from Netscape for the use in the Netscape Navigator browser, and Java is a "compiled" platform and browser independent general-purpose programming language from Sun.
One of the greatest problems nowadays still lies in the browser or platform dependence of the interactive programming. Java is supported by all relevant browsers, but it's not supported on Windows 3.1 systems, a fact that significantly reduces the "installed basis" and thus the number of potential respondents of Java-based questionnaires. JavaScript is supported on all platforms within the Netscape Navigator browser (version 2.0 and above), but other browsers do not or only partially (Microsoft Internet Explorer 3.0) support JavaScript.
Most of the following examples are based on JavaScript version 1.1 (as implemented in Netscape Navigator 3.0 or later (McComb 1996)) or the Java Development Kit version 1.02 from Sun (Sun Microsystems: Java Online Documentation). The examples are available on the Internet at (http://www.akzept.com).
3.1 Event Handling with JavaScript
In a questionnaire form (as a special case of a HTML-form), JavaScript statements are connected via "event handlers" to standardised HTML form elements such as buttons, text fields and listboxes. Those event handlers can recognise and respond to user input (events) such as mouse clicks and text input. The JavaScript code that controls the response of the HTML-element to user input is defined or called within the event handlers.
The objects used by JavaScript are mostly objects of the Browser itself (such as browser windows, forms and HTML-elements). Most of these objects have their own event handlers. Table 1 shows the most important event handlers for elements of HTML-forms (for details please refer to the JavaScript documentation of Netscape, an introduction is available from Stefan Koch (1996)).
Table 1 Event handlers of form elements
event applies to ... is activated ...
handler ...
onClick button, checkbox, when an object on a form is clicked.
radio, link, reset,
submit
onChange select, text, textarea when a select, text, or textarea field
loses focus and its value has been
modified.
onBlur select, text, textarea when a select, text or textarea field
loses focus.
onFocus select, text, textarea when a field receives input focus by
tabbing with the keyboard or clicking
with the mouse.
onMouseOut area, link each time the mouse pointer leaves an
area (client-side image map) or link
from inside that area or link.
onMouseOver area each time the mouse pointer moves over
an object area.
on Select text,textarea when a user selects some of the text
within a text or textarea field.
onSubmit form when the form is submitted.
The following code example makes extensive use of events. It shows the HTML- and JavaScript code for a "hybrid" question with two predefined answer categories (radiobuttons) and one "other" category with a radiobutton and a text field. The example uses images to visually "enlarge" the small radiobuttons. Each image "button" is linked via a hyperlink to the corresponding radiobutton. Whenever the respondent moves the mouse over the image the "mouseOver"-event occurs and a message is displayed in the status bar at the bottom of the browser window. When the picture button is clicked, the "click"-event for the corresponding radiobutton is initiated and the radiobutton is selected.
This allows for a clear and self-explaining design of buttons that are easier to activate than the small standard checkboxes and radiobuttons. Moreover, the researcher is able to turn arbitrary images (e.g. products, persons, logos) into clickable "buttons". In addition to the activation of buttons, the hyperlink element can be used to route the respondent to the next appropriate question after clicking one of the "buttons". To avoid inconsistencies in the data between the state of the radiobuttons and the open-ended "other" category, the content of the textfield is deleted whenever the respondent selects one of the predefined answer categories.
Code Example 1
<A HREF="JavaScript:void(0)" onMouseOver="self.status='Click Me'; return true"
onMouseOut="self.status=' '; return true"
onClick="self.document.testform.connect[0].click();
self.document.testform.other.value=''">
<IMG SRC="b_rb.GIF" HEIGHT=40 WIDTH=40></A>
<INPUT NAME="connect" TYPE="radio" VALUE="1"
onClick="self.document.testform.other.value=''">
Online Services (e.g. T-Online/AOL/Compuserve/Microsoft Network ...) <BR>
<A HREF="JavaScript:void(0)" onMouseOver="self.status='Click Me'; return true"
onMouseOut="self.status=' '; return true"
onClick="self.document.testform.connect[1].click();
self.document.testform.other.value=''">
<IMG SRC="b_rb.GIF" HEIGHT=40 WIDTH=40></A>
<INPUT name="connect" TYPE="radio" VALUE="2"
OnClick="self.document.testform.other.value=''">
Internet Service Provider <BR>
<A HREF="JavaScript:void(0)" onMouseOver="self.status='Click Me'; return true"
onMouseOut="self.status=' '; return true"
onClick="self.document.testform.elements[2].click();
self.document.testform.other.focus()">
<IMG SRC="b_rb.GIF" HEIGHT=40 WIDTH=40></A>
<INPUT name="connect" TYPE="radio" VALUE="3">
Others, please specify:
<INPUT NAME="other" TYPE="text" MAXLENGTH="60" SIZE="60"
onChange="self.document.testform.elements[2].click()">
In the following example an image is defined as an "imagemap". It's five regions respond to mouse-clicks and call the corresponding JavaScript commands defined in the imagemap. The image shows a 5 point scale and a text field to which the selected scale value is assigned. The researcher may select as an image whatever is most appropriate for the scaling task: scale values, descriptive text or iconized pictures. Moreover, this technique allows for the definition of an arbitrary image as an imagemap and the assignment of response values to any region of the image.
Code Example 2
pleasant<IMG USEMAP="#scalemap" SRC="scale5.GIF" ALT="scale 5" HEIGHT=30 WIDTH=150>unpleasant
<INPUT TYPE="text" NAME="scaleval" VALUE="?" SIZE="2" MAXLENGTH="1">
<INPUT TYPE="button" NAME="clear" VALUE="Clear" onClick="self.document.testform.scaleval.value=' '">
<MAP NAME="scalemap">
<AREA NAME="1" COORDS="1,0,30,30"
HREF="javascript:self.document.testform.elements['scaleval'].value='1'; void(0)">
<AREA NAME="2" COORDS="31,0,60,30"
HREF="javascript:self.document.testform.elements['scaleval'].value='2'; void(0)">
<AREA NAME="3" COORDS="61,0,90,30"
HREF="javascript:self.document.testform.elements['scaleval'].value='3'; void(0)">
<AREA NAME="4" COORDS="91,0,120,30"
HREF="javascript:self.document.testform.elements['scaleval'].value='4'; void(0)">
<AREA NAME="5" COORDS="121,0,150,30"
HREF="javascript:self.document.testform.elements['scaleval'].value='5'; void(0)">
</MAP>
3.2 The Use of Predefined and User-Defined Functions in Java Script
In addition to the "direct" call of JavaScript commands within event handlers, it is possible to define JavaScript functions within the HTML code of the questionnaire. The functions which can receive and return arguments have to be enclosed by the "SCRIPT"-Tag. The use of functions allows for an extremely flexible programming of standard routines for data handling and input validation.
In the following example the user defined function "validateNumber" represents a general routine for the validation of numeric input. This function checks for the existence of input, for non-numeric characters, and for the range of a given number. The function can be called from any number of text fields with different parameters that control the specific behaviour of the function. In the following example "validateNumber" is called from the "onBlur" event handler of the text field "number". The parameters passed to the function control which value is to be checked ("this"), the valid range of the input (15 to 25), whether a value is required (1=yes, 0=no), and a text for an error message. Error messages use the "alert" function of JavaScript, which opens a dialog box with the specified error message.
Code Example 3
<SCRIPT LANGUAGE="JavaScript">
function validateNumber(control, min, max, required, msg) {
msg = "\n" + msg + ": " + control.value;
var str = "" + control.value;
if (required && str.length == 0) {
alert(msg + "\nPlease enter a value !" ); control.focus(); return false;
}
if (!required && str.length == 0) {
return true;
}
for (var i = 0; i < str.length; i++) {
var ch = str.substring(i, i + 1);
if ((ch < "0" || "9" < ch) && ch != '.' ) {
alert(msg + "\nNot a numeric value. Please enter decimal numbers with a
".\"");
control.focus(); return false;
}
}
var num = 0 + str;
if (num < min || max < num) {
alert(msg + "\nThis value is not within the range [" + min + ".." + max + "].
");
control.focus(); return false;
}
}
</SCRIPT>
...
Please enter a number within the range of 15 to 25:
<INPUT TYPE="TEXT" NAME="number" SIZE=10 onBlur="validateNumber(this,15,25,1,'Number Input')">
<!--------------- Assign date to hidden field ------------------------->
<INPUT TYPE="hidden" NAME="datefield"> // hidden field
<SCRIPT LANGUAGE="JavaScript">
var date = new Date(); // JS date object is created ..
self.document.testform.datefield.value=date; // .. and assigned to the field
</SCRIPT>
...
In the above example the system variable "date" is assigned to a "hidden" field. The hidden fields are not displayed in the form but nevertheless they are submitted to the server.
3.3 Dynamic Creation of Browser Windows and Form Content with Java Script
JavaScript enables the researcher to create texts and HTML documents "on the fly". This allows the researcher to break down longer questionnaires into smaller and more manageable pages, to skip over irrelevant questions and to allow for a better control of the sequence in which the respondent has to answer the questions. Another major advantage of this technique is that even large and complex questionnaires can be "programmed" within the JavaScript code of one single HTML page. After loading this page the respondent may disconnect from the network and complete the questions without time pressure.
The following code example demonstrates only the "core" of this technique. While loading the main document, an array of strings (qArray) is created. The JavaScript function "makeScale5" preformats each item as a 5 point scale. The main form contains only one button which calls the function q(). This function opens up a new browser window called "quWin", generates a randomised array of items and writes the resulting HTML form to the new browser window.
Code Example 4
<SCRIPT LANGUAGE="JavaScript1.1">
var qArray = new Array();
function makeScale5(ItemName, Item) {
var t;
t = "<TABLE><TR><TD WIDTH='33%'>" + Item + "</TD>"
+ "<TD WIDTH='17%'><INPUT TYPE='text' NAME='"+ItemName+"' VALUE='' SIZE='2' ></TD>"
+ "<TD WIDTH='50%'>"
+ "<input type='Button' value=' 1 ' onClick='self.document.forms.testform."
+ ItemName + ".value = \"1\"'>"
+ "<input type='Button' value=' 2 ' onClick='self.document.forms.testform."
+ ItemName + ".value = \"2\"'>"
+ "<input type='Button' value=' 3 ' onClick='self.document.forms.testform."
+ ItemName + ".value = \"3\"'>"
+ "<input type='Button' value=' 4 ' onClick='self.document.forms.testform."
+ ItemName + ".value = \"4\"'>"
+ "<input type='Button' value=' 5 ' onClick='self.document.forms.testform."
+ ItemName + ".value = \"5\"'>"
+ "<input type='Button' value='Clear' onClick='self.document.forms.testform."
+ ItemName + ".value = \"\"'>"
+ "</TD></TR></TABLE>";
return t;
}
qArray[0] = makeScale5("qi1" , "text of item 1")
qArray[1] = makeScale5("qi2" , "text of item 2")
qArray[2] = makeScale5("qi3" , "text of item 3")
function randomizeItems(ItemArray) {
var t=""; var normArray = new Array(); var randArray = new Array();
for (i = 0; i < ItemArray.length; i++) {
var RandomNumber = Math.random();
normArray[" " + RandomNumber] = ItemArray[i];
randArray[i] = RandomNumber;
}
randArray.sort();
for (i = 0; i < ItemArray.length; i++) {
t = t + normArray[" "+ randArray[i]] + "<BR>"
}
return t;
}
function q() {
quWin = window.open(" ", "QuestionWindow",
"toolbar=no,width=550,height=400,directories=no,status=no,
scrollbars=yes,resize=no,menubar=no")
if (quWin != null) {
if (quWin.opener == null) {
quWin.opener = self;
}}
quWin.document.write("<FORM NAME='testform'><H4>text of question</H4>")
quWin.document.write(randomizeItems(qArray))
quWin.document.write("<HR><CENTER><INPUT TYPE='button' VALUE='Close'
onClick='self.close();
quWin=null;'></CENTER></FORM>")
}
</SCRIPT>
</HEAD><BODY BGCOLOR="#FFFFFF">
<H2 ALIGN=CENTER>Sample 5: Dynamic Generation of Windows</H2>
<FORM NAME="testform" >
<CENTER><INPUT TYPE="button" VALUE="Go!" onClick="eval(q())"></CENTER>
</FORM></BODY></HTML>
3.4 Interaction of JavaScript and Java: Basics and Application
The above code examples show an increasing complexity of program code. To reduce the time and programming skills necessary for questionnaire design and to make the code more reusable it makes sense to build libraries of tried and tested, flexible questionnaire components such as form elements, complete predefined question templates, routines for input validation and data management as "building blocks" for further questionnaire programming.
The Java language allows for the creation of programs that can be executed within a browser window (applets). Unlike JavaScript, Java is a browser and platform independent programming language. Major disadvantages to the use of Java lie in the fact that Java it is not supported (for technical reasons) on Windows 3.1x platforms and that the interface between Java applets and forms/browser objects is still browser-dependent.
The LiveConnect feature of Netscape Navigator 3.0 makes all public variables, methods, and properties of an applet available for JavaScript access. This feature allows for the implementation of commonly used routines in Java applets which then serve as function libraries. Moreover, Java applets can be used to create new form elements. To access JavaScript functions from a Java applet, it is necessary to import a Netscape-specific Java code into the applet's program code. In addition to this, the author of an HTML page must permit an applet to access JavaScript by specifying the MAYSCRIPT attribute of the APPLET tag.
A simple example of the use of Java-to-JavaScript communication is available at http://www.akzept.com. It is a variation of the previous JavaScript example. In the example an applet replaces the "Go"-button and the JavaScript function "makeScale5". The following line of code includes the applet "livecon.class" under the name "LiveCon" in the main document of the browser.
<APPLET CODE="livecon.class" NAME="LiveCon" WIDTH="160" HEIGHT="70" MAYSCRIPT></APPLET>
The following function call replaces the function call to the JavaScript function "makeScale5" of the previous example.
qArray[0] = document.LiveCon.makeScale5("qi1" , "text of item 1")
4. Conclusion
As already pointed out, a full platform- and browser independence of interactive questionnaire programming is not yet possible. This may (hopefully) change in the near future with new technologies such as Java Beans. For the time being it is important to keep the questionnaire as complex as necessary and as simple as possible. To ignore this (apparently trivial but difficult to fulfill) demand means to endanger one's reputation in the Internet.
References
Batinic,Bernad (1996), 'Die Durchführung von Fragebogenuntersuchungen im INTERNET - ein erster Überblick. Version 3.0 (5.9.1996)',
http://www.psychol.uni-giessen.de/~Batinic/survey/frag_faq.htm
Batinic, Bernad (1996), 'Sonstige Untersuchungen im Internet',
http://www.psychol.uni-giessen.de/~Batinic/survey/fra_andr.htm
Hippler, H.J.; (1988). 'Methodische Aspekte schriftlicher Befragungen: Probleme und Forschungsperspektiven'. Planung und Analyse 6/88, 244-248.
Jacobs, Bernhard (1996), 'Fragebogen erstellen und auswerten im WWW'
http://www.phil.uni-sb.de/FR/Medienzentrum/verweise/psych/wwwfrage/ wwwfrage.html
Koch, Stefan (1996), 'Introduction to Javascript',
http://rummelplatz.uni-mannheim.de/~skoch/js/index.htm
McComb, G. (1996). 'New JavaScript features in Navigator 3.0', JavaWorld October 1996,
http://www.javaworld.com/javaworld/jw-10-1996/jw-10-javascript.htm.
Netscape Communications Corporation (1996), 'The JavaScript Guide',
http://home.netscape.com/eng/mozilla/3.0/handbook/javascript/toc.html
Romppe, Matthias (1996), 'Untersuchungen im Internet',
http://www.gwdg.de/~mromppe/is5.htm
Sun Microsystems Inc: JavaSoft, Java Online Documentation,
http://java.sun.com/java.sun.com /newdocs.html
Netscape Navigator and JavaScript are trademarks of Netscape Communications Corporation.
Java is a trademark of Sun Microsystems, Inc.
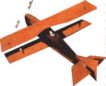
Anfragen und Informationsaustausch sind jederzeit willkommen:
Friedrich@Schuster.net
Bitte verwenden Sie für Bookmarks die URL
"http://Friedrich.Schuster.net"
Copyright (c) 1995 - 2000 F. Schuster. All Rights Reserved.
[ Top ]
[ Contact ]
|